Gravity Simulation Modeling gravitational force with MATLAB
I’ve done all types of projects, but I figured I’d save the best for first. I’ve created a gravity simulation to model planet interaction, and it’s my favorite coding project that I’ve done so far.
In this post, I’ll go through my basic design process and some of the physics and programming I used to get to a super cool simulation. Click here if you’d rather skip straight to the final simulation. Click here it you’d like to check out my code on github.
Project Background
This past summer, I was a tutor for the first ever UCLA Engineering Transfer Summer Bridge program, designed to help students transferring into UCLA’s engineering program get a head-start on their courses and get a feel for the rigor of the curriculum.
We introduced students to MATLAB, a programming language commonly used by engineers and researchers for all kinds of things, including processing data and running simulations. The final project of this two-week crash-course was to create a simulation of gravity. This simulation could be used to model all kinds of space-y things, from calculating how to launch a satellite into orbit around Mars, or modelling the formation of planets from a bunch of space dust. It also introduced students into some programming concepts like iteration and data structures.
As a tutor, I helped formulate the project idea and problem statement. I also got to build my own version of the gravity simulation as an example. I’ll go through the physics and math that drives this simulation, as well as the programming tips and tricks used in this project.
Gravity
Gravity is an attractive force between objects thats proportional to their mass and inversely proportional to the square of the distance between them. Basically, the bigger you are the more you attractive you are; the further away you are, the less attractive you are. You can read more about it here, but it’s best summed up by this equation:
F_k,j = [G*m_j*m_k / ||dx||³] * dx
||dx|| = √(dx² + dy²+ dz²)
where F_k,j is the force of attraction between body k and body j, G is the universal gravitational constant, m_k is the mass of the first object, m_j is the mass of the second object and dx is the separation vector between the two bodies. The ||…|| notation denotes the Euclidean norm of a vector; it’s a way to calculate the magnitude of the vector, which in this case, is the distance between the two objects.
Kinematics
With this equation, we know how much each object is pulling on each other. This pulling force (F) is going to make these objects move; how fast they move (acceleration a) depends on their size (mass m). This is summarized in the equation:
F = m*a
We can then find how fast the object is going (velocity v) and calculate where it will be (position x) after some given time (differential time step dt). This is summarized with the equations:
a = v* dt
v = x*dt.
Basically, we can use gravity to predict where the planets will be at some tiny amount of time in the future. But now they’ve moved, which means the gravitational force between the two is different! We need to recalculate the gravitational force between the objects, and then figure out the new acceleration, velocity and position, over and over until we’re done with our experiment.
That’s a lot of calculations to do by hand, especially if we want to add more planets. But computers are really good at doing these calculations for us. Next, I’ll go over how I wrote a program in MATLAB to create this simulation, which perform these calculations and display the results.
Collisions
Lastly we need to figure out what to do when object collide. We’re going to model our collision as an inelastic collision, where the two objects will accrete, or combine into a single object with a combined mass and new velocity. In these types of collisions, momentum of the system is conserved. We can use the equation:
m_1*v_1 + m_2*v_2 = m_new*v_new
We know that m_new = m_1 + m_2, since the new object is the combined masses of the two objects. We rearrange and solve for v_new:
v_new = (m_1*v_1 + m_2*v_2) / (m_1 + m_2)
Now we know the new object’s mass and velocity, and can treat it like any other object in our system.
*Bolded variables are vectors
Building the Program
Basics
A computer is actually pretty simple; it follows whatever instructions it is given. A set of instructions is called an algorithm. Programmers create these sets of instructions that the computer follows. These instructions are first written in human language (like English), so the human programmer can easily understand what’s going on. We call this human version of the program pseudocode. Then we convert that into code, the language the computer understands.
*From now on, I refer to objects as bodies since not everything in space is a planet (r.i.p. Pluto), but you can think of them as planets if you’d like.
Here’s the pseudocode for the gravity simulation:
Create all the bodies in the system, including their mass, starting velocity and position
Repeat what’s in {} for every instant for the duration of the simulation:
{ Check for collisions between bodies
Calculate the gravitational force between each pair of bodies
Calculate the new velocity and position of each body }
This is what we described we’d need to do earlier! You can check out my code on github to see how this is translated into MATLAB so the computer can understand.
Verification
Once we’ve written our code, it’s important to verify that it’s working the way we expect. I used a simple case of two equal-sized bodies at rest. The two bodies will attract each other until they collide and become one, stationary body, shown here:
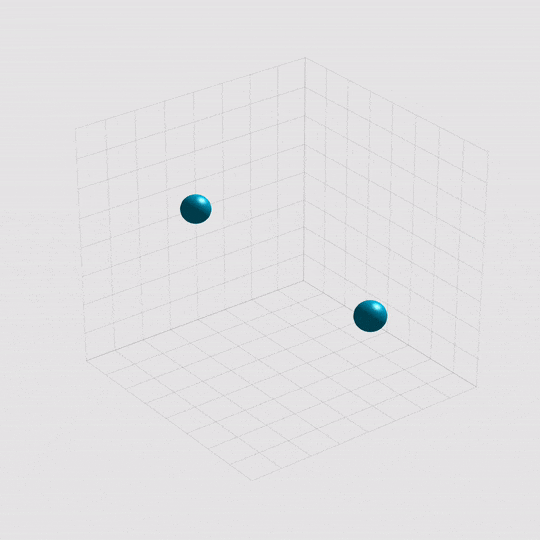
This simple case verified a lot of things: the bodies moved toward each other, so I’d gotten the force calculation correct; the bodies combined into one, so I’m detecting collisions correctly; the bodies stopped moving, so I’m conserving momentum. Now that I know the basic part of my code is working, I can add more complicated features.
Special behavior: Explosions
So far, we’re modeling an inelastic collision, where the objects combine when they collide. But what happens when two big objects collide head-on? We’d expect them to explode, with pieces shooting out from the point of collision. But so far, we’ve only modeled accretion.
To model an explosion, we calculate how much energy was involved in the collision. If the collision is powerful enough, the bodies will explode instead of combining, as seen here
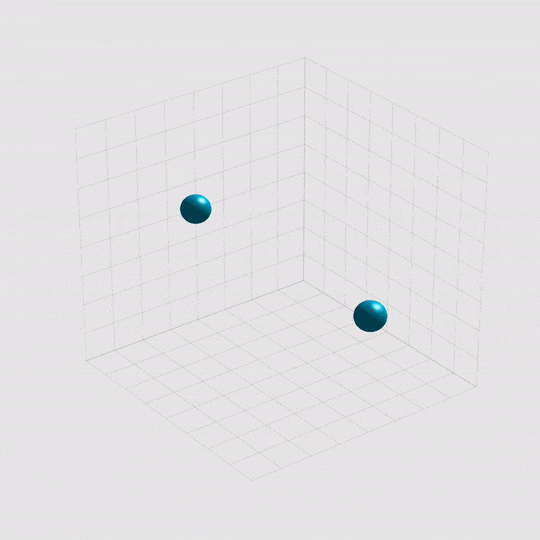
Gravity Simulation Demo
I’ve picked a few interesting examples of the gravity simulation. All these demos are start with bodies randomly placed in a small area. All bodies are a similar size with small random starting velocities. The color of of the bodies depends on its mass. The smaller objects are blue and bigger objects are red.
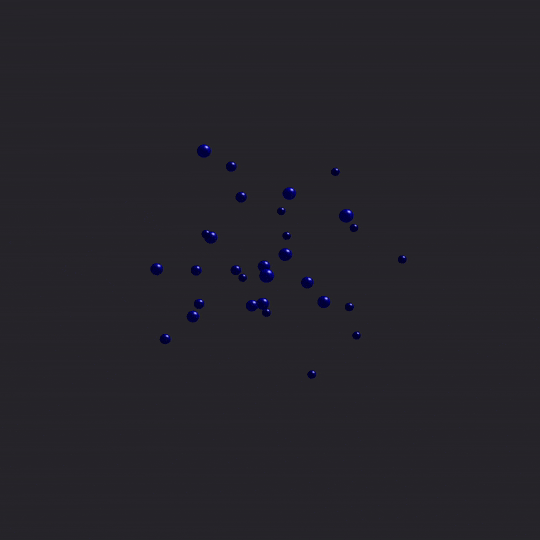
You can see it’s rather satisfying to watch how the chaotic system evolves. You can also see that a simple principle can be used to create a complicated simulation.
Final Thoughts
I’ve got a few ideas for where I want to take this project. My next goal is to develop a web-based version of this simulation, so users can run a new simulation every time, with control over all the different setting. I also plan to do more research into explosions to make the fragmentation feature more realistic.
If you wanna learn to code yourself, there’s a whole bunch of stuff online to help you get started (that don’t cost money like MATLAB). I started out with Codecacdemy and recommend it to all beginners.
You can find the code for this simulation on my github; you can also check out this project and others in my portfolio.
I’ve got plenty more projects to showcase: stay tuned for more!